ran slicing
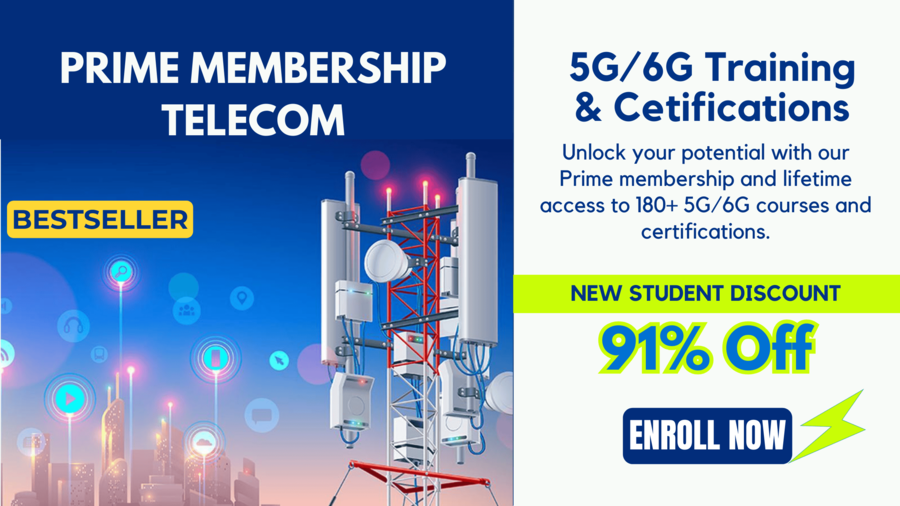
Range slicing typically refers to extracting a portion (a range) of elements from a sequence, such as a list or a string, based on indices. In Python, you can use the slice notation to achieve range slicing. The syntax for slicing is as follows:
pythonCopy codesequence[start:stop:step]
start
: The index of the first element you want to include in the slice (inclusive).stop
: The index of the first element you don't want to include in the slice (exclusive).step
: The step or stride between elements in the slice. It is optional and defaults to 1.
Here's an example with a list:
pythonCopy codemy_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9
]slice_result = my_list[2:7] # This will extract elements at indices 2, 3, 4, 5, and 6
(slice_result)
print
The output will be:
pythonCopy code[2, 3, 4, 5, 6
]
In this example, my_list[2:7]
extracts a slice starting from index 2 (inclusive) up to index 7 (exclusive).
You can also use negative indices for reverse slicing:
pythonCopy codereverse_slice = my_list[-5:-2] # This will extract elements at indices -5, -4, and -3
(reverse_slice)
print
The output will be:
pythonCopy code[5, 6, 7
]
Keep in mind that if you omit start
, stop
, or step
in the slice notation, it will default to the entire sequence, and you can use negative indices to count from the end of the sequence.